4.8.2.13. TIRE_FORCE
This subroutine defines a user - defined tire force.
Language type |
Subroutine |
FORTRAN |
tire_usub (TIME, ITIME, CONPROP, TIREPROP, MASSPROP, UPAR, NPAR, JFLAG, IFLAG, TFSAE, RFSAE, FCOEF) |
C/C++ |
tire_usub (double time, double itime, double conprop[], double tireprop[], double massprop[], double upar[], int npar, int jflag, int iflag, double tfsae[3], double rfsae[3], double fcoef[2]) |
Variable Name |
Size |
Description |
time |
double |
Current simulation time of RecurDyn/Solver. |
itime |
double |
Past time for saved values. |
conprop |
double[10] |
Contact property for computing Tire force.
|
tireprop |
double[11] |
Geometry and Material property of TIRE.
|
massprop |
double[2] |
Mass property of TIRE. |
upar |
double[100] |
Parameters defined by the user. The maximum size of array is 100. |
npar |
int |
Number of user parameters. |
jflag |
int |
When RecurDyn/Solver evaluates the Jacobian, the flag is true. Otherwise, the flag is false. |
iflag |
int |
When RecurDyn/Solver makes its initial call to this routine, the flag is true. Otherwise, the flag is false. |
tfsae |
double[3] |
Returned force vector acting at the contact point in the SAE coordinate system and this is three dimensional arrays.
|
rfsae |
double[3] |
Returned torque vector acting at the contact point in the SAE coordinate system this is three dimensional arrays.
|
fcoef |
double[2] |
Returned friction coefficients this is two dimensional arrays.
|
Example
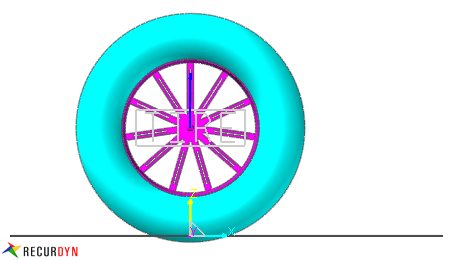
Figure 4.117 Tire model
Note
If the user wants to run this model using a user subroutine, the user can refer it in the directory (<install dir>\Help\usub\**).
C---- SUB. TIRE_FORCE
SUBROUTINE TIRE_FORCE
& (TIME,ITIME,CONPROP,TIREPROP,MASSPROP,UPAR,NPAR,
& JFLAG,IFLAG,TFSAE,RFSAE,FCOEF)
C---- TO EXPORT * SUBROUTINE
!DEC$ ATTRIBUTES DLLEXPORT,C::TIRE_FORCE
C---- INCLUDE SYSTEM CALL
INCLUDE 'SYSCAL.F'
C---- DEFINE VARIABLES
C Parameter Information
C TIME : Simulation time of RD/Solver. (Input)
C ITIME : Past time for saved values. (Input)
C CONPROP : Contact property for computung Tire force. (Input)
C TIREPROP : Geometry and Material property of TIRE. (Input)
C MASSPROP : Mass property of TIRE. (Input)
C UPAR : Parameters defined by user. (Input)
C NPAR : Number of user parameters. (Input)
C JFLAG : When RD/Solver evaluates a Jacobian, the flag is true. (Input)
C IFLAG : When RD/Solver initializes arrays, the flag is true. (Input)
C TFSAE : Returned force vector acting at the contact point in the SAE coordinate system. (Output, Size : 3)
C RFSAE : Returned torque vector acting at the contact point in the SAE coordinate system. (Output, Size : 3)
C FCOEF : Returned friction coefficients. (Output, Size : 2)
DOUBLE PRECISION TIME, ITIME, UPAR(*)
DOUBLE PRECISION CONPROP(*), TIREPROP(*), MASSPROP(*)
INTEGER NPAR
LOGICAL JFLAG, IFLAG
DOUBLE PRECISION TFSAE[REFERENCE](3), RFSAE[REFERENCE](3)
DOUBLE PRECISION FCOEF[REFERENCE](2)
C---- USER STATEMENT
DOUBLE PRECISION VALUE1
DOUBLE PRECISION zkForce, zcForce, new_zcForce
DOUBLE PRECISION ver_stiff, pen, mass, damping, dot_pen, max_pen
LOGICAL errflg
c step function
pen = CONPROP(4)
max_pen = 0.01* TIREPROP(2)
CALL rd_step(pen, 0, 0, max_pen, 1, 1, VALUE1, errflg)
c CACLULATION FOR NORMAL FORCE
ver_stiff = TIREPROP(3);
zkForce = -ver_stiff*pen;
mass = MASSPROP(1)
damping = TIREPROP(8)
dot_pen = CONPROP(5)
new_zcForce = -2.0*sqrt(mass*abs(ver_stiff))*damping*dot_pen
zcForce = VALUE1*new_zcForce
c Z AXIS NORMAL FORCE
TFSAE(3) = min(0.0, zkForce+zcForce)
RETURN
END
#include "stdafx.h"
#include "DllFunc.h"
#include "math.h"
TireForce_API void __cdecl tire_force
(double time, double itime, double conprop[], double tireprop[], double massprop[],
double upar[], int npar, int jflag, int iflag, double tfsae[3], double rfsae[3], double fcoef[2])
{
using namespace rd_syscall;
// Parameter Information
// time : Simulation time of RD/Solver. (Input)
// itime : Past time for saved values. (Input)
// conprop : Contact property for computung Tire force. (Input)
// tireprop : Geometry and Material property of TIRE. (Input)
// massprop : Mass property of TIRE. (Input)
// upar : Parameters defined by user. (Input)
// npar : Number of user parameters. (Input)
// jflag : When RD/Solver evaluates a Jacobian, the flag is true. (Input)
// iflag : When RD/Solver initializes arrays, the flag is true. (Input)
// tfsae : Returned force vector acting at the contact point in the SAE coordinate system. (Output, Size : 3)
// rfsae : Returned torque vector acting at the contact point in the SAE coordinate system. (Output, Size : 3)
// fcoef : Returned friction coefficients. (Output, Size : 2)
// User Statement
double value;
double zkForce, zcForce, new_zcForce;
double ver_stiff, pen, mass, damping, dot_pen, max_pen;
int errflg;
//step function
pen = conprop[3];
max_pen = 0.01* tireprop[1];
rd_step(pen, 0, 0, max_pen, 1, 1, &value, &errflg);
//calculation for normal force
ver_stiff = tireprop[2];
zkForce = -ver_stiff*pen;
mass = massprop[0];
damping = tireprop[7];
dot_pen = conprop[4];
new_zcForce = -2.0*sqrt(mass*abs(ver_stiff))*damping*dot_pen;
zcForce = value*new_zcForce;
//z axis normal force
tfsae[2] = min(0.0, zkForce+zcForce);
}